In this tutorial, I will show you how to load a URDF File into RViz. Universal Robot Description Format (URDF) is the standard ROS format for robot modeling.
Prerequisites
- ROS 2 Foxy Fitzroy installed on Ubuntu Linux 20.04
- If you are using another ROS 2 distribution, you will need to replace ‘foxy’ with the name of your distribution everywhere I mention ‘foxy’ in this tutorial.
- I highly recommend you get the newest version of ROS 2. If you are using a newer version of ROS 2, you can still follow all the steps in this tutorial.
- You have already created a ROS 2 workspace. The name of our workspace is “dev_ws”, which stands for “development workspace.”
You can find the entire code for this project here on my Google Drive.
What is URDF?
A URDF (Universal Robot Description Format) file is an XML file that describes what a robot should look like in real life. It contains the complete physical description of the robot.
You can learn more about URDF files here.
Install Important ROS 2 Packages
First, we need to install some important ROS 2 packages that we will use in this tutorial. Open a new terminal window, and type the following commands, one right after the other.
sudo apt install ros-foxy-joint-state-publisher-gui
sudo apt install ros-foxy-xacro
The format of the commands above is:
sudo apt install ros-<ros2-distro>-joint-state-publisher-gui
sudo apt install ros-<ros2-distro>-xacro
You will need to replace <ros2-distro> with the ROS 2 distribution you are using. In this case, I am using ROS 2 Foxy Fitzroy, which is ‘foxy’ for short.
Create a ROS 2 Package
Let’s create a ROS 2 package inside our workspace. My workspace is named dev_ws.
In a new terminal window, move to the src (source) folder of your workspace.
cd ~/dev_ws/src
Now create the package using the following command.
ros2 pkg create --build-type ament_cmake two_wheeled_robot
Create Extra Folders
Move inside the package.
cd ~/dev_ws/src/two_wheeled_robot/
Create some extra folders with the following names. We will not use all of these folders. I just like to have these folders in every package I create in case I need to use them.
mkdir config launch maps meshes models params rviz urdf worlds
Type the following command to verify the folders were created.
dir
Now build the package by typing the following command:
cd ~/dev_ws
colcon build
Let’s add a script to our bashrc file which enables us to use the following command to move to the package from any directory inside our terminal window.
colcon_cd two_wheeled_robot
Where ‘cd’ means “change directory”.
Note that in ROS 1, we typed roscd to change directories. In ROS 2, we use the colcon_cd command instead of roscd.
Open a terminal window, and type the following commands, one right after the other:
echo "source /usr/share/colcon_cd/function/colcon_cd.sh" >> ~/.bashrc
echo "export _colcon_cd_root=~/dev_ws" >> ~/.bashrc
Now open a new terminal window.
To go directly to the basic_mobile_robot ROS 2 package, type:
colcon_cd two_wheeled_robot
This command above will get you straight to the two_wheeled_robot package from within any directory in your Linux system. The format is:
colcon_cd [ROS 2 package name]
Create the URDF File
In this section, we will build our mobile robot step-by-step. Our robot will be defined in a Universal Robot Descriptor File (URDF), the XML file that represents a robot model.
Open a new terminal window, and type:
colcon_cd two_wheeled_robot
cd urdf
Make sure you have the Gedit text editor installed.
sudo apt-get install gedit
Create a new file named two_wheeled_robot.urdf.
gedit two_wheeled_robot.urdf
Inside this file, we define what our robot will look like (i.e. visual properties), how the robot will behave when it bumps into stuff (i.e. collision properties), and its mass (i.e. inertial properties).
Type this code inside the URDF file.
Save and close the file.
Now go to the meshes folder.
cd ~/dev_ws/src/two_wheeled_robot/meshes
Add this STL file to your meshes folder. A mesh is a file that allows your robot to look more realistic (rather than just using basic shapes like boxes and spheres).
Rather than use the STL files I provided, you can use a program like SolidWorks to generate your own STL files. You can also use a program called Blender to create DAE files. URDF files support meshes in either STL or DAE format.
Add Dependencies
Let’s add some packages that our project will depend on. Go to the package.xml file.
cd ~/dev_ws/src/two_wheeled_robot/
OR
colcon_cd two_wheeled_robot
gedit package.xml
After the <buildtool_depend> tag, add the following lines:
<exec_depend>joint_state_publisher</exec_depend>
<exec_depend>robot_state_publisher</exec_depend>
<exec_depend>rviz</exec_depend>
<exec_depend>xacro</exec_depend>
Save the file, and close it.
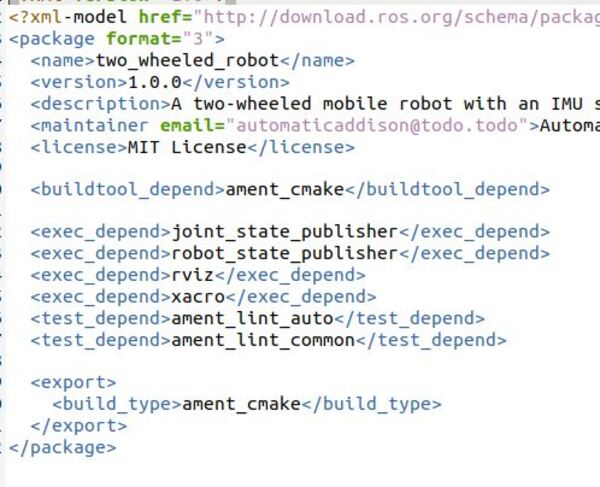
We will be using the Joint State Publisher and the Robot State Publisher. We will also be using RViz to visualize our robot model.
Create the Launch File
Let’s create a launch file. Open a new terminal window, and type:
colcon_cd two_wheeled_robot
cd launch
gedit two_wheeled_robot.launch.py
Copy and paste this code into the file.
# Author: Addison Sears-Collins
# Date: September 14, 2021
# Description: Launch a two-wheeled robot URDF file using Rviz.
# https://automaticaddison.com
import os
from launch import LaunchDescription
from launch.actions import DeclareLaunchArgument
from launch.conditions import IfCondition, UnlessCondition
from launch.substitutions import Command, LaunchConfiguration
from launch_ros.actions import Node
from launch_ros.substitutions import FindPackageShare
def generate_launch_description():
# Set the path to this package.
pkg_share = FindPackageShare(package='two_wheeled_robot').find('two_wheeled_robot')
# Set the path to the RViz configuration settings
default_rviz_config_path = os.path.join(pkg_share, 'rviz/rviz_basic_settings.rviz')
# Set the path to the URDF file
default_urdf_model_path = os.path.join(pkg_share, 'urdf/two_wheeled_robot.urdf')
########### YOU DO NOT NEED TO CHANGE ANYTHING BELOW THIS LINE ##############
# Launch configuration variables specific to simulation
gui = LaunchConfiguration('gui')
urdf_model = LaunchConfiguration('urdf_model')
rviz_config_file = LaunchConfiguration('rviz_config_file')
use_robot_state_pub = LaunchConfiguration('use_robot_state_pub')
use_rviz = LaunchConfiguration('use_rviz')
use_sim_time = LaunchConfiguration('use_sim_time')
# Declare the launch arguments
declare_urdf_model_path_cmd = DeclareLaunchArgument(
name='urdf_model',
default_value=default_urdf_model_path,
description='Absolute path to robot urdf file')
declare_rviz_config_file_cmd = DeclareLaunchArgument(
name='rviz_config_file',
default_value=default_rviz_config_path,
description='Full path to the RVIZ config file to use')
declare_use_joint_state_publisher_cmd = DeclareLaunchArgument(
name='gui',
default_value='True',
description='Flag to enable joint_state_publisher_gui')
declare_use_robot_state_pub_cmd = DeclareLaunchArgument(
name='use_robot_state_pub',
default_value='True',
description='Whether to start the robot state publisher')
declare_use_rviz_cmd = DeclareLaunchArgument(
name='use_rviz',
default_value='True',
description='Whether to start RVIZ')
declare_use_sim_time_cmd = DeclareLaunchArgument(
name='use_sim_time',
default_value='True',
description='Use simulation (Gazebo) clock if true')
# Specify the actions
# Publish the joint state values for the non-fixed joints in the URDF file.
start_joint_state_publisher_cmd = Node(
condition=UnlessCondition(gui),
package='joint_state_publisher',
executable='joint_state_publisher',
name='joint_state_publisher')
# A GUI to manipulate the joint state values
start_joint_state_publisher_gui_node = Node(
condition=IfCondition(gui),
package='joint_state_publisher_gui',
executable='joint_state_publisher_gui',
name='joint_state_publisher_gui')
# Subscribe to the joint states of the robot, and publish the 3D pose of each link.
start_robot_state_publisher_cmd = Node(
condition=IfCondition(use_robot_state_pub),
package='robot_state_publisher',
executable='robot_state_publisher',
parameters=[{'use_sim_time': use_sim_time,
'robot_description': Command(['xacro ', urdf_model])}],
arguments=[default_urdf_model_path])
# Launch RViz
start_rviz_cmd = Node(
condition=IfCondition(use_rviz),
package='rviz2',
executable='rviz2',
name='rviz2',
output='screen',
arguments=['-d', rviz_config_file])
# Create the launch description and populate
ld = LaunchDescription()
# Declare the launch options
ld.add_action(declare_urdf_model_path_cmd)
ld.add_action(declare_rviz_config_file_cmd)
ld.add_action(declare_use_joint_state_publisher_cmd)
ld.add_action(declare_use_robot_state_pub_cmd)
ld.add_action(declare_use_rviz_cmd)
ld.add_action(declare_use_sim_time_cmd)
# Add any actions
ld.add_action(start_joint_state_publisher_cmd)
ld.add_action(start_joint_state_publisher_gui_node)
ld.add_action(start_robot_state_publisher_cmd)
ld.add_action(start_rviz_cmd)
return ld
Save the file, and close it.
You can read more about Launch files here.
Add the RViz Configuration File
Let’s add a configuration file that will initialize RViz with the proper settings so we can view the robot as soon as RViz launches.
Open a new terminal window. Type:
colcon_cd two_wheeled_robot
cd rviz
gedit rviz_basic_settings.rviz
Add this code.
Save the file, and close it.
Build the Package
Now we need to build the package. Open a new terminal window, and type:
colcon_cd two_wheeled_robot
gedit CMakeLists.txt
Add the following snippet to CMakeLists.txt file above the if(BUILD_TESTING) line.
install(
DIRECTORY config launch maps meshes models params rviz src urdf worlds
DESTINATION share/${PROJECT_NAME}
)
Save the file and close it.
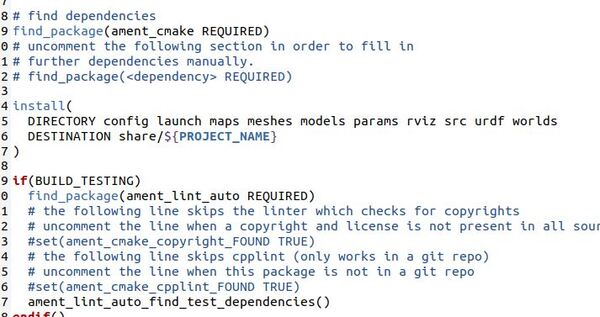
Build the project.
cd ~/dev_ws/
colcon build
In the future, if you would like to build the two_wheeled_robot package only, you can type:
colcon build --packages-select two_wheeled_robot
Launch the Robot in RViz
Open a new terminal, and launch the robot.
cd ~/dev_ws/
ros2 launch two_wheeled_robot two_wheeled_robot.launch.py
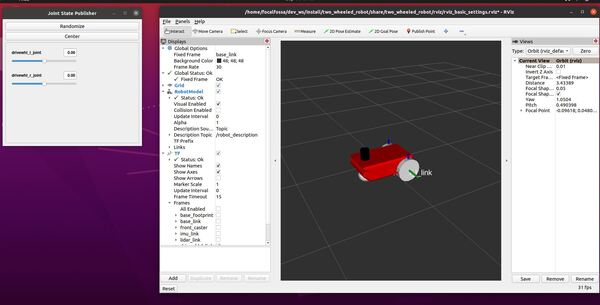
——
By the way, if you want to see the available arguments you can pass to the launch file from the terminal window, type:
ros2 launch -s two_wheeled_robot two_wheeled_robot.launch.py
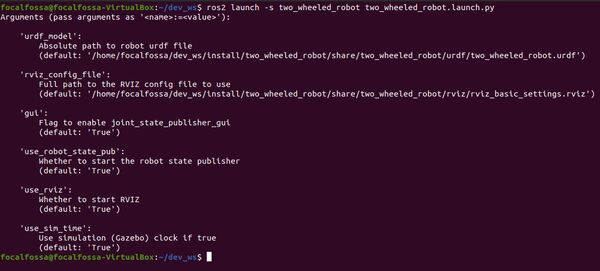
And if you want to set the value of an argument (e.g. launch the robot without RViz), you can do something like this:
ros2 launch two_wheeled_robot two_wheeled_robot.launch.py use_rviz:='False'
——
Here is the output after we launch the robot in RViz:
The joint state publisher GUI has sliders that enable you to move the wheels.
If something goes wrong with the launch, close everything down, restart Linux, and launch again.
Check whether you have properly set up the collision properties by enabling Collision Enabled under RobotModel on the left pane.
You can see in the image that I have modeled the base_link as a box for collisions. I could have just as easily used the STL model itself (which was used for visualization) as the collision geometry, but I decided to go with the simpler box representation to give me more flexibility to alter the collision areas if necessary. Also, Gazebo (a popular robotics simulator) recommends using simpler collision geometry to increase performance.
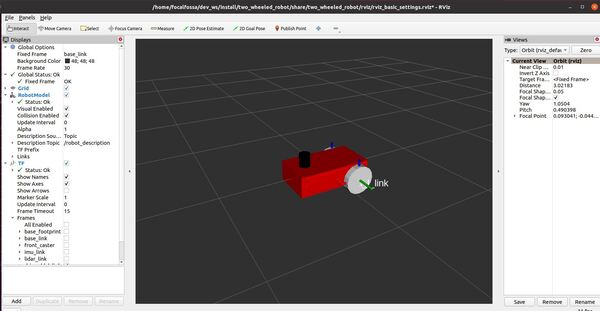
View the Coordinate Frames
Let’s see the coordinate frames. We first need to install the necessary software.
sudo apt install ros-foxy-tf2-tools
Check out the coordinate frames.
colcon_cd two_wheeled_robot
ros2 run tf2_tools view_frames.py
As of ROS Galactic, this command is:
ros2 run tf2_tools view_frames
In the current working directory, you will have a file called frames.pdf. Open that file.
evince frames.pdf
Here is what my coordinate transform (i.e. tf) tree looks like:
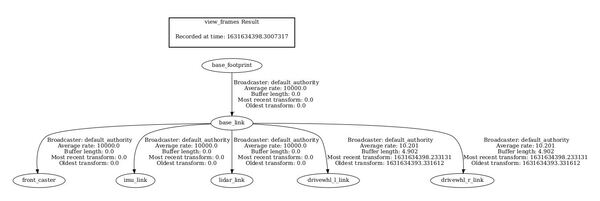
If we want to see the coordinate transformation from one link to another, we can type the following command. For example, what is the position and orientation of the front caster wheel relative to the base_link of the robot?
The syntax is:
ros2 run tf2_ros tf2_echo <parent frame> <child frame>
We open a terminal window, and type:
ros2 run tf2_ros tf2_echo base_link front_caster
Here is the output. With respect to the base_link reference frame, the front caster wheel is located at (x=0.217 meters, y=0 meters, and z=-0.1 meters).

The rotation value is in quaternion format. You can see that the rotation values are all 0s, indicating that the orientation of both coordinate frames is equivalent (i.e. the x, y, and z axes of both coordinate frames point in the same direction).
Remember that, by convention:
- x-axis is red
- y-axis is green
- z-axis is blue
To see an image of the architecture of our ROS system, open a new terminal window, and type the following command:
rqt_graph
Select the “Nodes/Topics (all) option in the upper-left part of the screen, and then click the refresh arrow right next to it.
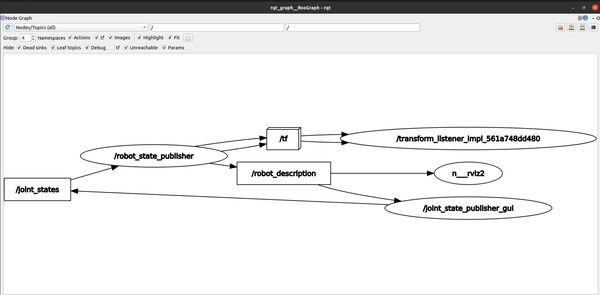
You can see how the joint state publisher GUI manipulates the joint states (i.e. the angle of each wheel joint). This data feeds into the robot state publisher. The robot state publisher publishes the coordinate frame relationships to tf, and the updated robot model feeds into RViz, the robot model visualization program.
That’s it!
When you’re done, press CTRL+C in all terminal windows to shut everything down.