In this tutorial, I will show you how to send a simulated robot (in Gazebo) to goal locations using ROS.
Real-World Applications
This project has a number of real-world applications:
- Indoor and Outdoor Delivery Robots
- Room Service Robots
- Robot Vacuums
- Order Fulfillment
- Manufacturing
- Factories
Our robot will exist in a world that contains a post office and three houses. The use case for this simulated robot would be picking up packages at a post office and delivering them to houses in a neighborhood.
Let’s get started!
Prerequisites
- You have completed this tutorial where you learned how to create a simulated mobile manipulator.
- You have completed this tutorial where you set up and configured the ROS Navigation Stack for your robot.
- All the code you need is located at this link.
Getting Started
In our Gazebo environment, we have four main goal locations we would like to send our robot.
- 1 – House 1
- 2 – House 2
- 3 – House 3
- 4 – Post Office
Open a new terminal window, and launch the robot in Gazebo.
roslaunch mobile_manipulator mobile_manipulator_gazebo.launch
Then in another terminal window, type:
roslaunch mobile_manipulator mobile_manipulator_move_base.launch
Make a note of the X and Y coordinates of each desired goal location. I use the RViz Point Publish button to accomplish this. When you click that button, you can see the coordinate values by typing the following command in a terminal:
ros topic echo /clicked_point
I want to have an X, Y coordinate for the following four goal locations in the world.
1 = House 1 (-15.04, -7.42, 0.0)
2 = House 2 (-14.25, 20.02, 0.0)
3 = House 3 (7.35, 20.17, 0.0)
4 = Post Office (12.12, -8.41, 0.0)
You notice how I numbered the goal locations above. That was intentional. I want to be able to type a number into a terminal window and have the robot navigate to that location.
For example, if I type 4, the robot will move to the post office. If I type 2, the robot will go to house 2.
Write the Code
Now let’s write some C++.
Open a terminal window.
roscd mobile_manipulator/src
gedit send_goals.cpp
Add this code.
Save the file, and close it.
Compile the Code
Now open a new terminal window, and type the following command:
roscd mobile_manipulator
gedit CMakeLists.txt
Go to the bottom of the file.
Add the following lines.
INCLUDE_DIRECTORIES(/usr/local/lib)
LINK_DIRECTORIES(/usr/local/lib)
add_executable(send_goals src/send_goals.cpp)
target_link_libraries(send_goals ${catkin_LIBRARIES})
cd ~/catkin_ws/
Compile the package.
catkin_make --only-pkg-with-deps mobile_manipulator
Run the Code
Open a new terminal window, and launch the robot in Gazebo.
roslaunch mobile_manipulator mobile_manipulator_gazebo.launch
Then in another terminal window, type:
roslaunch mobile_manipulator mobile_manipulator_move_base.launch
Open another terminal to launch the send_goals node.
rosrun mobile_manipulator send_goals
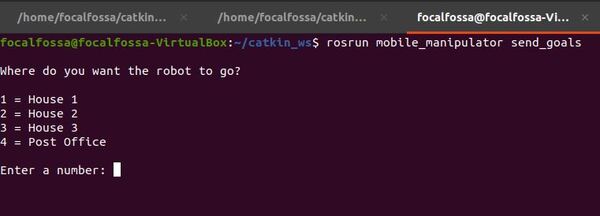
Follow the prompt to send your goal to the ROS Navigation Stack.
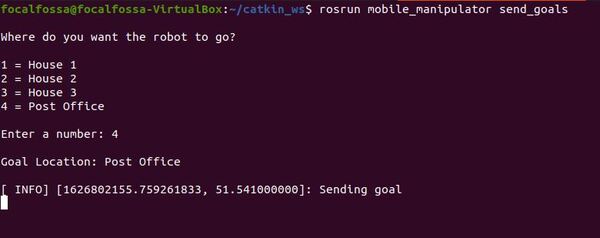
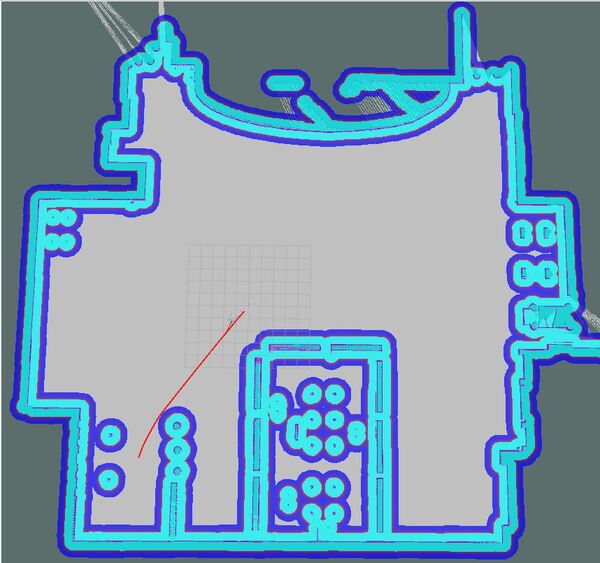
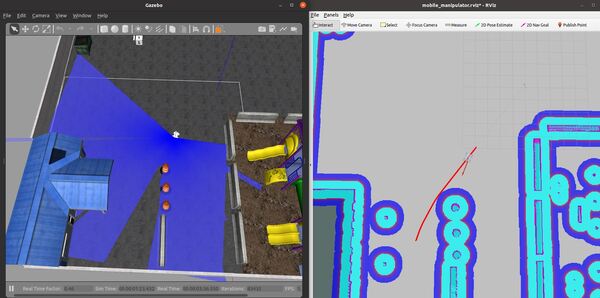
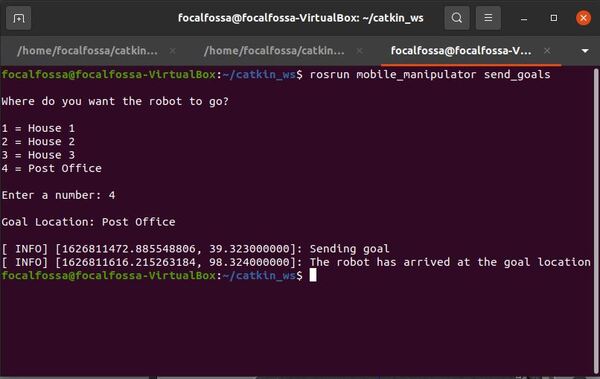
To see the node graph (which shows what ROS nodes are running to make all this magic happen), type:
rqt_graph
If the robot is having trouble reaching the goal, you can adjust the xy_goal_tolerance (in meters) and the yaw_goal_tolerance (in radians) parameters inside the base_local_planner_params.yaml file, which is located in my ~/catkin_ws/src/mobile_manipulator/param folder.
That’s it. Keep building!