In this tutorial, I will show you how to create an ArUco Marker from scratch using OpenCV (Python). I will follow this tutorial.
If you do a search online for “aruco marker generator”, you can find a lot of free ArUco marker generators online. Here is one. We will create our own ArUco generator though.
Prerequisites
- You have OpenCV (Python) installed on your system (pip install opencv-contrib-python).
- (Optional) You are able to run the code on this tutorial without any problems.
Create the Code
Open your favorite code editor, and write the following code. I will name my program generate_aruco_marker.py. This program generates an ArUco marker and saves it to your computer.
#!/usr/bin/env python
'''
Welcome to the ArUco Marker Generator!
This program:
- Generates ArUco markers using OpenCV and Python
'''
from __future__ import print_function # Python 2/3 compatibility
import cv2 # Import the OpenCV library
import numpy as np # Import Numpy library
# Project: ArUco Marker Generator
# Date created: 12/17/2021
# Python version: 3.8
# Reference: https://www.pyimagesearch.com/2020/12/14/generating-aruco-markers-with-opencv-and-python/
desired_aruco_dictionary = "DICT_ARUCO_ORIGINAL"
aruco_marker_id = 1
output_filename = "DICT_ARUCO_ORIGINAL_id1.png"
# The different ArUco dictionaries built into the OpenCV library.
ARUCO_DICT = {
"DICT_4X4_50": cv2.aruco.DICT_4X4_50,
"DICT_4X4_100": cv2.aruco.DICT_4X4_100,
"DICT_4X4_250": cv2.aruco.DICT_4X4_250,
"DICT_4X4_1000": cv2.aruco.DICT_4X4_1000,
"DICT_5X5_50": cv2.aruco.DICT_5X5_50,
"DICT_5X5_100": cv2.aruco.DICT_5X5_100,
"DICT_5X5_250": cv2.aruco.DICT_5X5_250,
"DICT_5X5_1000": cv2.aruco.DICT_5X5_1000,
"DICT_6X6_50": cv2.aruco.DICT_6X6_50,
"DICT_6X6_100": cv2.aruco.DICT_6X6_100,
"DICT_6X6_250": cv2.aruco.DICT_6X6_250,
"DICT_6X6_1000": cv2.aruco.DICT_6X6_1000,
"DICT_7X7_50": cv2.aruco.DICT_7X7_50,
"DICT_7X7_100": cv2.aruco.DICT_7X7_100,
"DICT_7X7_250": cv2.aruco.DICT_7X7_250,
"DICT_7X7_1000": cv2.aruco.DICT_7X7_1000,
"DICT_ARUCO_ORIGINAL": cv2.aruco.DICT_ARUCO_ORIGINAL
}
def main():
"""
Main method of the program.
"""
# Check that we have a valid ArUco marker
if ARUCO_DICT.get(desired_aruco_dictionary, None) is None:
print("[INFO] ArUCo tag of '{}' is not supported".format(
args["type"]))
sys.exit(0)
# Load the ArUco dictionary
this_aruco_dictionary = cv2.aruco.Dictionary_get(ARUCO_DICT[desired_aruco_dictionary])
# Allocate memory for the ArUco marker
# We create a 300x300x1 grayscale image, but you can use any dimensions you desire.
print("[INFO] generating ArUCo tag type '{}' with ID '{}'".format(
desired_aruco_dictionary, aruco_marker_id))
# Create the ArUco marker
this_marker = np.zeros((300, 300, 1), dtype="uint8")
cv2.aruco.drawMarker(this_aruco_dictionary, aruco_marker_id, 300, this_marker, 1)
# Save the ArUco tag to the current directory
cv2.imwrite(output_filename, this_marker)
cv2.imshow("ArUco Marker", this_marker)
cv2.waitKey(0)
if __name__ == '__main__':
print(__doc__)
main()
Save the file, and close it.
You need to have opencv-contrib-python installed and not opencv-python. Open a terminal window, and type:
pip uninstall opencv-python
pip install opencv-contrib-python
To run the program in Linux for example, type the following command:
python3 generate_aruco_marker.py
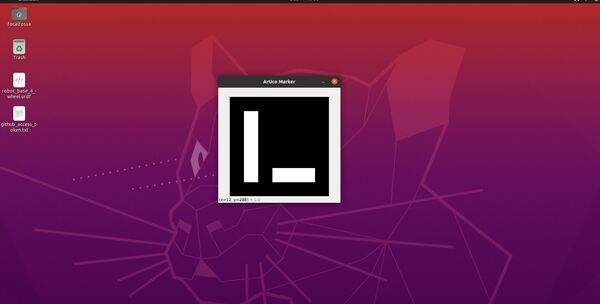
If you want to restore OpenCV to the previous version after you’re finished creating the ArUco markers, type:
pip uninstall opencv-contrib-python
pip install opencv-python
To set the changes, I recommend rebooting your computer.
That’s it! Keep building!