In this tutorial, we’ll learn how to create a ROS package. Software in ROS is organized into packages. Each package might contain a mixture of code (e.g. ROS nodes), data, libraries, images, documentation, etc. Every program you write in ROS will need to be inside a package.
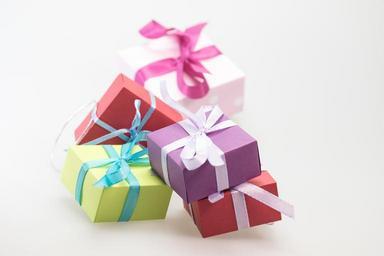
ROS packages promote software reuse. The goal of a ROS package is to be large enough to provide a specific useful functionality, but not so large and complicated that nobody wants to reuse it for their own project.
ROS packages are organized as follows:
- launch folder: Contains launch files
- src folder: Contains the source code (C++, Python)
- CMakeLists.txt: List of cmake rules for compilation
- package.xml: Package information and dependencies
Here is the official ROS tutorial on how to create a ROS package. I will walk you through this process below.
Directions
Here is the syntax for creating a ROS package. Do not run this piece of code.
catkin_create_pkg <package_name> [depend1] [depend2] [depend3]
package_name is the name of the package you want to make, and depend1, depend2, depend3, etc., are the names of other ROS packages that your package depends on.
Now, open a new terminal window, and move to the source directory of the workspace you created. If you don’t already have a workspace set up, check out this tutorial.
cd ~/catkin_ws/src
Create a ROS package named ‘hello_world’ inside the src folder of the catkin workspace (i.e. catkin_ws). This package will have three dependencies (i.e. libraries the package depends on in order for the code inside the package to run properly): roscpp (the ROS library for C++), rospy (the ROS library for Python), and std_msgs (common data types that have been predefined in ROS … “standard messages”).
catkin_create_pkg hello_world std_msgs rospy roscpp
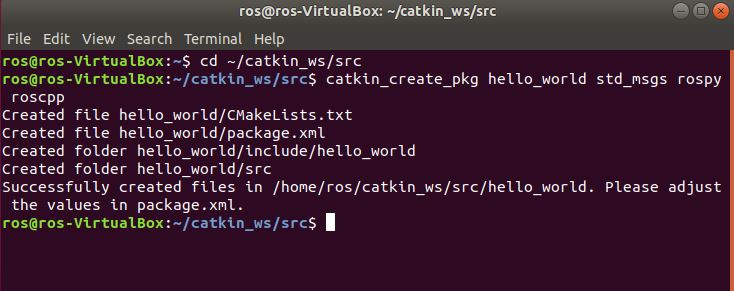
Type dir , and you will see that we now have a package named hello_world inside the source folder of the workspace.
Change to the hello_world package.
cd hello_world
Note, we could have also used the ROS command to move to the package.
roscd hello_world
Type:
dir
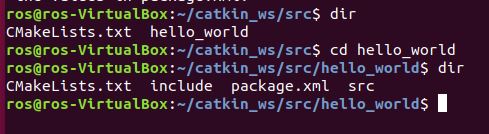
You can see that we have four files:
CMakeLists.txt: This text file contains the commands to compile the programs that you write in ROS. It also has the commands to convert your source code and other files into an executable (i.e. the code that your computer can run).
include: Contains package header files. You might remember when we wrote header files in the C++ tutorial…well this folder is where your header files would be stored.
src: This folder will contain the C++ source code. If you are doing a project in Python, you can create a new folder named scripts that will contain Python code. To create this new folder type:
mkdir scripts

package.xml: This is an Extensible Markup Language (XML) file. An XML file is a text file written in a language (called XML) that is easy to read by both humans and computers. An XML file does not do anything other than store information in a structured way.
Our package.xml file contains information about the hello_world package. You can see what is inside it by typing:
gedit package.xml
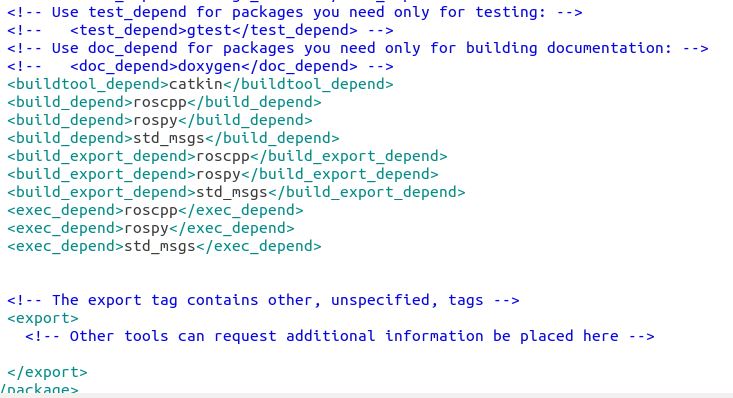
That’s it! You’re all done.
Note that if you ever want to go straight to a package without typing the full path, ROS has a command to enable you to do that. In the terminal window, type the following:
roscd <package_name>
For example, if we want to go straight to the hello_world package, we would open a new terminal window and type the following command:
roscd hello_world
Compiling a Package in ROS
When you create a new package in ROS, you need to compile it in order for it to be able to run. The command to compile a package in ROS is as follows:
catkin_make
The command above will compile all the stuff inside the src folder of the package.
You must be inside your catkin_ws directory in order for the catkin_make command to work. If you are outside the catkin_ws directory, catkin_make won’t work.
To get to your catkin_ws directory, you can type this command anywhere inside your terminal:
roscd
cd ..
Then to compile all the packages inside the catkin workspace, you type:
catkin_make
If you just want to compile specific packages (in cases where your project is really big), you type this command:
catkin_make --only-pkg-with-deps
For example, if you have a package named hello_world, you can compile just that package:
catkin_make --only-pkg-with-deps hello_world