In this tutorial, we will explore arrays, vectors, and strings using C++.
Prerequisites
- You have completed this tutorial: How to Use Operators and Control Flow in C++.
- I am assuming you are using Visual Studio Code, but you can use any code editor.
Managing Arrays
Let’s explore how to manage arrays in C++ for robotics. Arrays are a fundamental data structure that allows you to store and manipulate multiple values of the same type.
Open a terminal window, and type this:
cd ~/Documents/cpp_tutorial
code .
Let’s create a new C++ file and name it robot_arrays.cpp.
Type the following code into the editor:
#include <iostream>
using namespace std;
int main() {
const int size = 5;
int sensor_readings[size];
// Initializing the array
for (int i = 0; i < size; i++) {
sensor_readings[i] = i * 10;
}
// Accessing array elements
cout << "Sensor Readings:" << endl;
for (int i = 0; i < size; i++) {
cout << "Sensor " << i + 1 << ": " << sensor_readings[i] << endl;
}
return 0;
}
In this example, we demonstrate how to declare, initialize, and access elements of an array.
First, we declare a constant size to represent the size of the array. Then, we declare an integer array sensor_readings with a size of size.
Next, we use a for loop to initialize the array elements. In this case, we assign each element a value that is a multiple of 10 based on its index.
After initializing the array, we use another for loop to access and print each element of the array. We use the array indexing syntax sensor_readings[i] to access individual elements, where i is the index of the element.
Run the code.
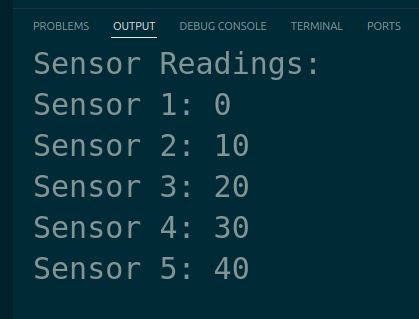
You should see the sensor readings printed in the terminal.
This example demonstrates the basic management of arrays in C++ for robotics. Arrays are useful for storing and processing collections of related data, such as sensor readings, robot positions, or any other data that needs to be organized and accessed efficiently.
When working with arrays, it’s important to be mindful of the array size and ensure that you don’t access elements outside the valid range to avoid undefined behavior.
Arrays are a powerful tool in robotic programming, allowing you to store and manipulate data efficiently. They are commonly used for tasks such as storing sensor data, tracking robot positions, or representing configuration parameters.
Employing Vectors
Let’s explore how to employ vectors in C++ for robotics. Vectors are a dynamic array container provided by the C++ Standard Library that offer flexibility and convenience when working with resizable arrays.
Let’s create a new C++ file and name it robot_vectors.cpp.
Type the following code into the editor:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> motor_speeds;
// Adding elements to the vector
motor_speeds.push_back(100);
motor_speeds.push_back(200);
motor_speeds.push_back(150);
// Accessing vector elements
cout << "Motor Speeds:" << endl;
for (int i = 0; i < motor_speeds.size(); i++) {
cout << "Motor " << i + 1 << ": " << motor_speeds[i] << endl;
}
return 0;
}
In this example, we demonstrate how to declare, add elements to, and access elements of a vector.
First, we include the <vector> header to use the vector container. Then, we declare a vector named motor_speeds that will store integer values.
To add elements to the vector, we use the push_back() member function. In this case, we add three motor speed values to the vector.
To access the elements of the vector, we use a for loop that iterates from 0 to the size of the vector.
We use the size() member function to get the number of elements in the vector. Inside the loop, we access each element using the array indexing syntax motor_speeds[i], where i is the index of the element.
Run the code.
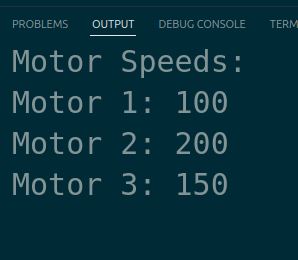
You should see the motor speeds printed in the terminal.
In robotic projects, you can use vectors to store and process sensor readings, track robot trajectories, manage lists of tasks, or any other scenario where dynamic arrays are needed.
Manipulating Strings
Let’s explore how to manipulate strings in C++ for robotics. Strings are a fundamental data type used to represent and work with text data.
Let’s create a new C++ file and name it robot_strings.cpp.
Type the following code into the editor:
#include <iostream>
#include <string>
using namespace std;
int main() {
string robot_name = "AutomaticAddisonBot";
// Accessing string characters
cout << "Robot Name: " << robot_name << endl;
cout << "First Character: " << robot_name[0] << endl;
// Modifying string
robot_name[8] = '-';
cout << "Modified Robot Name: " << robot_name << endl;
// Concatenating strings
string model = "X100";
string full_name = robot_name + " " + model;
cout << "Full Name: " << full_name << endl;
return 0;
}
In this example, we demonstrate how to declare, access, modify, and concatenate strings.
First, we include the <string> header to use the string data type.
Then, we declare a string variable named robot_name and assign it the value “AutomaticAddisonBot”.
To access individual characters of the string, we use the array indexing syntax. We print the first character of the robot name using robot_name[0].
To modify a character in the string, we use the array indexing syntax and assign a new value to a specific position. In this case, we change the character at index 8 to a hyphen.
To concatenate strings, we use the + operator. We declare a new string variable model and assign it the value “X100”.
Then, we concatenate robot_name, a space character, and model to create a new string full_name, which represents the complete name of the robot.
Run the code.
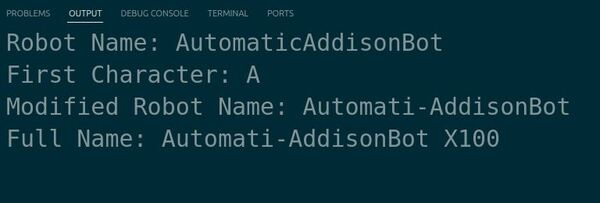
You should see the robot name, the first character, the modified robot name, and the full name printed in the terminal.
This example demonstrates the basic manipulation of strings in C++ for robotics. Strings are essential for working with text data, such as robot names, commands, or messages.
Employing Character Functions
Let’s explore how to employ character functions in C++ for robotics. Character functions are useful for manipulating and analyzing individual characters in a string.
Let’s create a new C++ file and name it robot_char_functions.cpp.
Type the following code into the editor:
#include <iostream>
#include <cctype>
using namespace std;
int main() {
char command = 'f';
// Checking character properties
if (islower(command)) {
cout << "Command is lowercase" << endl;
}
// Converting character case
char uppercase_command = toupper(command);
cout << "Uppercase Command: " << uppercase_command << endl;
// Checking character equality
if (command == 'f') {
cout << "Command is 'f'" << endl;
}
return 0;
}
In this example, we demonstrate how to use character functions to check character properties, convert character case, and compare characters.
First, we include the <cctype> header to use the character functions.
Then, we declare a character variable command and assign it the value ‘f’.
To check if a character is lowercase, we use the islower() function. It returns a non-zero value if the character is lowercase, and zero otherwise.
We use an if statement to check the result and print a message if the command is lowercase.
To convert a character to uppercase, we use the toupper() function. It returns the uppercase equivalent of the character. We assign the result to a new character variable uppercase_command and print it.
To compare characters, we use the equality operator ==. In this case, we check if the command is equal to the character ‘f’. If the condition is true, we print a message indicating that the command is ‘f’.
Run the code.
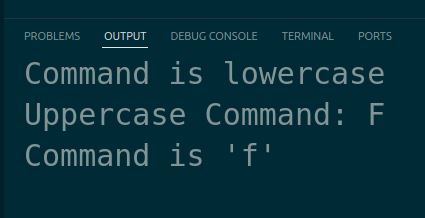
You should see the messages indicating that the command is lowercase, the uppercase equivalent of the command, and that the command is ‘f’ printed in the terminal.
In robotic projects, you can use character functions for tasks such as validating user input, processing command strings, or analyzing sensor data that involves individual characters.
Keep building!