In this tutorial, we will explore how to use the std namespace in C++ for robotics. The std namespace helps organize your code and makes it more readable.
Prerequisites
- You have completed this tutorial: How to Run C++ Code That Uses External Libraries.
- I am assuming you are using Visual Studio Code, but you can use any code editor.
Directions
Open a terminal window, and type this:
cd ~/Documents/cpp_tutorial && code .
Let’s create a new C++ file and name it simple_robot.cpp.
Type the following code into the editor:
#include <iostream>
#include <string>
using namespace std;
int main() {
string robot_name = "AutomaticAddisonBot";
int robot_battery = 80;
cout << "Robot Name: " << robot_name << endl;
cout << "Battery Level: " << robot_battery << "%" << endl;
return 0;
}
In this code, we include the iostream and string headers for input/output operations and string manipulation, respectively.
We use the using namespace std; statement to bring the std namespace into the current scope, allowing us to use elements like cout, endl, and string without the std:: prefix.
Inside the main function, we declare variables for robot_name and robot_battery, representing the name and battery level of the robot.
We print the robot’s name and battery level using cout and endl without the std:: prefix since we are using the std namespace.
Run the code.
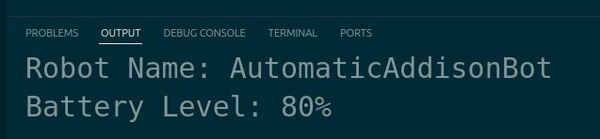
You should see the robot’s name and battery level printed in the terminal.
This example demonstrates how using the std namespace can make your code more concise and readable.
Thanks, and I’ll see you in the next tutorial.
Keep building!