In this tutorial, we will create a C++ publisher for ROS 2. Knowing how to write a publisher node is one of the most important skills in robotics software engineering.
In ROS 2 (Robot Operating System 2), a C++ publisher is a program (written in C++) that sends messages across the ROS network to other parts of the system.
The official instructions for creating a publisher are here, but I will walk you through the entire process, step by step.
We will be following the ROS 2 C++ Style Guide.
Follow along with me click by click, keystroke by keystroke.
Let’s get started!
Prerequisites
- You have completed this tutorial: How to Create a ROS 2 Python Publisher – Jazzy.
- I am assuming you are using Visual Studio Code, but you can use any code editor.
Why C++?
So why should we write a publisher node in C++ instead of in Python?
Using C++ instead of Python for a ROS 2 node is like choosing a high-performance sports car over a regular sedan for a race. C++ is generally faster and more efficient, which is important for real-time applications where quick responses are necessary.
Also, C++ gives us better control over how the robot’s brain (the node) uses its resources, like memory and processing power.
And while Python is easier to work with, especially for beginners, C++ provides the speed and precision needed for complex tasks, making it the preferred choice for developing ROS 2 nodes in scenarios where performance is important.
Write the Code
Open a terminal, and type these commands to open VS Code.
cd ~/ros2_ws && code .
Right-click on the src/ros2_fundamentals_examples/src folder, and create a new file called “cpp_minimal_publisher.cpp”.
Type the following code inside cpp_minimal_publisher.cpp:
/**
* @file cpp_minimal_publisher.cpp
* @brief Demonstrates publishing string messages to a ROS 2 topic.
*
* Description: Demonstrates the basics of publishing messages within
* the ROS 2 framework.
*
* The core functionality of this publisher is to repeatedly send out
* string messages at a fixed frequency.
*
* -------
* Subscription Topics:
* None
* -------
* Publishing Topics:
* String message
* /cpp_example_topic - std_msgs/String
* -------
* @author Addison Sears-Collins
* @date November 5, 2024
*/
#include "rclcpp/rclcpp.hpp" // ROS 2 C++ client library
#include "std_msgs/msg/string.hpp" // Standard message type for strings
using namespace std::chrono_literals; // Handles time duration
/**
* @class MinimalCppPublisher
* @brief Defines a minimal ROS 2 publisher node.
*
* This class inherits from rclcpp::Node and demonstrates creating a
* publisher, publishing messages, and using a timer callback in ROS 2.
*/
class MinimalCppPublisher : public rclcpp::Node
{
public:
/**
* @brief Constructs a MinimalCppPublisher node.
*
* Sets up a publisher for 'std_msgs::msg::String' messages on
* the "/cpp_example_topic" topic and initializes a timer to call
* the timerCallback method.
*/
MinimalCppPublisher() : Node("minimal_cpp_publisher"), count_(0)
{
// Create a publisher object for sending string messages
// with a queue size of 10.
publisher_ = create_publisher<std_msgs::msg::String>(
"/cpp_example_topic", 10);
// Set up a timer to call the timerCallback function
timer_ = create_wall_timer(500ms,
std::bind(&MinimalCppPublisher::timerCallback, this));
RCLCPP_INFO(get_logger(), "Publishing at 2 Hz");
}
/**
* @brief Timer callback function.
*
* This method is called at a fixed interval.
* It publishes a string message
* containing "Hello World" followed by a sequence number.
*
* @return void
*/
void timerCallback()
{
// Create a new String message object.
auto message = std_msgs::msg::String();
message.data = "Hello World! " + std::to_string(count_++);
// Publish the message
publisher_->publish(message);
}
private:
// Member variables
size_t count_; // Keep track of the number of messages published
rclcpp::Publisher<std_msgs::msg::String>::SharedPtr
publisher_; // The publisher object
rclcpp::TimerBase::SharedPtr timer_; // Timer
};
/**
* @brief Main function.
*
* Initializes the ROS 2 system and runs the minimal_cpp_publisher node.
* It keeps the node alive until it is manually terminated.
*/
int main(int argc, char * argv[])
{
// Initialize ROS 2.
rclcpp::init(argc, argv);
// Create an instance of the MinimalCppPublisher node and keep it running.
auto minimal_cpp_publisher_node = std::make_shared<MinimalCppPublisher>();
rclcpp::spin(minimal_cpp_publisher_node);
// Shutdown ROS 2 upon node termination.
rclcpp::shutdown();
// End of program.
return 0;
}
Configure CMakeLists.txt
Now we need to modify the CMakeLists.txt file inside the package so that the ROS 2 system will be able to find the cost we just wrote.
Open up the CMakeLists.txt file that is inside the package.
Make it look like this:
cmake_minimum_required(VERSION 3.8)
project(ros2_fundamentals_examples)
# Check if the compiler being used is GNU's C++ compiler (g++) or Clang.
# Add compiler flags for all targets that will be defined later in the
# CMakeLists file. These flags enable extra warnings to help catch
# potential issues in the code.
# Add options to the compilation process
if(CMAKE_COMPILER_IS_GNUCXX OR CMAKE_CXX_COMPILER_ID MATCHES "Clang")
add_compile_options(-Wall -Wextra -Wpedantic)
endif()
# Locate and configure packages required by the project.
find_package(ament_cmake REQUIRED)
find_package(ament_cmake_python REQUIRED)
find_package(rclcpp REQUIRED)
find_package(rclpy REQUIRED)
find_package(std_msgs REQUIRED)
# Define a CMake variable named dependencies that lists all
# ROS 2 packages and other dependencies the project requires.
set(dependencies
rclcpp
std_msgs
)
# Add the specified directories to the list of paths that the compiler
# uses to search for header files. This is important for C++
# projects where you have custom header files that are not located
# in the standard system include paths.
include_directories(
include
)
# Tells CMake to create an executable target named cpp_minimal_publisher
# from the source file src/cpp_minimal_publisher.cpp. Also make sure CMake
# knows about the program's dependencies.
add_executable(cpp_minimal_publisher src/cpp_minimal_publisher.cpp)
ament_target_dependencies(cpp_minimal_publisher ${dependencies})
#add_executable(cpp_minimal_subscriber src/cpp_minimal_subscriber.cpp)
#ament_target_dependencies(cpp_minimal_subscriber ${dependencies})
# Copy necessary files to designated locations in the project
install (
DIRECTORY ros2_fundamentals_examples
DESTINATION share/${PROJECT_NAME}
)
install(
DIRECTORY include/
DESTINATION include
)
# Install cpp executables
install(
TARGETS
cpp_minimal_publisher
# cpp_minimal_subscriber
DESTINATION lib/${PROJECT_NAME}
)
# Install Python modules for import
ament_python_install_package(${PROJECT_NAME})
# Add this section to install Python scripts
install(
PROGRAMS
ros2_fundamentals_examples/py_minimal_publisher.py
DESTINATION lib/${PROJECT_NAME}
)
# Automates the process of setting up linting for the package, which
# is the process of running tools that analyze the code for potential
# errors, style issues, and other discrepancies that do not adhere to
# specified coding standards or best practices.
if(BUILD_TESTING)
find_package(ament_lint_auto REQUIRED)
# the following line skips the linter which checks for copyrights
# comment the line when a copyright and license is added to all source files
set(ament_cmake_copyright_FOUND TRUE)
# the following line skips cpplint (only works in a git repo)
# comment the line when this package is in a git repo and when
# a copyright and license is added to all source files
set(ament_cmake_cpplint_FOUND TRUE)
ament_lint_auto_find_test_dependencies()
endif()
# Used to export include directories of a package so that they can be easily
# included by other packages that depend on this package.
ament_export_include_directories(include)
# Generate and install all the necessary CMake and environment hooks that
# allow other packages to find and use this package.
ament_package()
Configure package.xml
Now we need to configure the package.xml file.
Open the package.xml file, and make sure it looks like this:
<?xml version="1.0"?>
<?xml-model href="http://download.ros.org/schema/package_format3.xsd" schematypens="http://www.w3.org/2001/XMLSchema"?>
<package format="3">
<name>ros2_fundamentals_examples</name>
<version>0.0.0</version>
<description>Basic examples demonstrating ROS 2 concepts</description>
<maintainer email="automaticaddison@example.com">Addison Sears-Collins</maintainer>
<license>Apache-2.0</license>
<!--Specify build tools that are needed to compile the package-->
<buildtool_depend>ament_cmake</buildtool_depend>
<buildtool_depend>ament_cmake_python</buildtool_depend>
<!--Declares package dependencies that are required for building the package-->
<depend>rclcpp</depend>
<depend>rclpy</depend>
<depend>std_msgs</depend>
<!--Specifies dependencies that are only needed for testing the package-->
<test_depend>ament_lint_auto</test_depend>
<test_depend>ament_lint_common</test_depend>
<export>
<build_type>ament_cmake</build_type>
</export>
</package>
Build the Workspace
To build the workspace, open a terminal window, and type:
build
If this command doesn’t work, type these commands:
echo "alias build='cd ~/ros2_ws/ && colcon build && source ~/.bashrc'" >> ~/.bashrc
build
Run the Node
Open a terminal window, and type:
ros2 run ros2_fundamentals_examples cpp_minimal_publisher
Let’s check out the list of topics.
ros2 topic list
Let’s see the data coming over the /cpp_example_topic topic.
ros2 topic echo /cpp_example_topic
Let’s see how often the topic is publishing a new message.
ros2 topic hz /cpp_example_topic
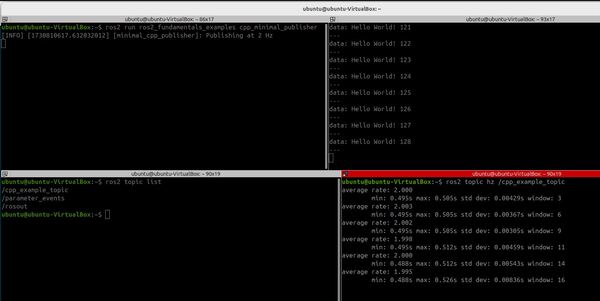
That’s it. I will see you in the next tutorial.
Keep building!