In this tutorial, we will explore how to use structs and operator overloading in C++.
Prerequisites
- You have completed this tutorial: How to Use Inheritance and Abstraction in C++.
- I am assuming you are using Visual Studio Code, but you can use any code editor.
Working with Structs
Let’s explore how to work with structs in C++ and their application in robotics projects.
Structs can be used in C++ to group related data together, making the code more organized and readable. Structs are particularly useful in robotics projects for representing various data structures, such as robot configurations, sensor readings, or control parameters.
Open a terminal window, and type this:
cd ~/Documents/cpp_tutorial
code .
Create a new C++ file named robot_struct.cpp.
Type the following code into the editor:
#include <iostream>
#include <string>
struct RobotSpec {
std::string model;
int max_speed;
double weight;
};
void print_robot_spec(const RobotSpec& spec) {
std::cout << "Model: " << spec.model << std::endl;
std::cout << "Max Speed: " << spec.max_speed << " m/s" << std::endl;
std::cout << "Weight: " << spec.weight << " kg" << std::endl;
}
int main() {
RobotSpec robot = {"MobileBot", 5, 10.5};
print_robot_spec(robot);
return 0;
}
In this code, we define a struct called RobotSpec to represent the specifications of a robot. It contains three members: model (a string), max_speed (an integer), and weight (a double).
We also define a function print_robot_spec() that takes a constant reference to a RobotSpec object and prints its members.
In the main() function, we create an instance of the RobotSpec struct called robot and initialize its members.
We then pass robot to the print_robot_spec() function to print its specifications.
Run the code.
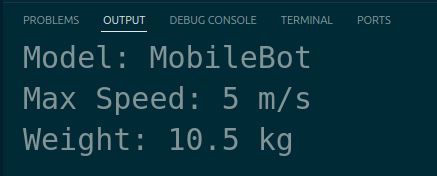
The output displays the robot’s specifications as defined in the RobotSpec struct.
Implementing Operator Overloading
Let’s explore the concept of operator overloading in C++ and its applications in robotics projects.
Operator overloading is a powerful feature in C++ that allows you to define custom behavior for operators when applied to user-defined types. This means you can make operators like +, -, *, /, and others work with your own classes and objects in a way that makes sense for your specific use case.
Let’s create a new C++ file named distance.cpp to demonstrate operator overloading.
Type the following code into the editor:
#include <iostream>
class Distance {
public:
int meters;
Distance(int m = 0) : meters(m) {}
Distance operator+(const Distance& other) const {
return Distance(meters + other.meters);
}
};
int main() {
Distance d1(5);
Distance d2(10);
Distance sum = d1 + d2;
std::cout << "d1 = " << d1.meters << " meters" << std::endl;
std::cout << "d2 = " << d2.meters << " meters" << std::endl;
std::cout << "sum = " << sum.meters << " meters" << std::endl;
return 0;
}
In this code, we define a class called Distance to represent a distance in meters. We overload the + operator by defining the operator+() member function. This function takes another Distance object as a parameter and returns a new Distance object that is the sum of the two distances.
In the main() function, we create two Distance objects, d1 and d2, and compute their sum using the overloaded + operator. We then print the distances and their sum using the meters member variable.
Run the code.
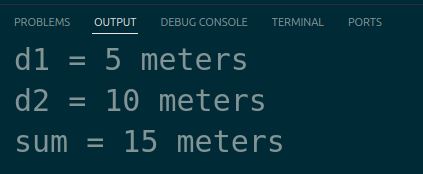
The output displays the distances d1 and d2, as well as their sum, in meters.
By overloading the + operator, we’ve made it possible to add two Distance objects together using the familiar syntax d1 + d2, which makes the code more intuitive and readable.
In robotics projects, operator overloading can be useful for performing operations on custom data types, such as distances, angles, or time durations, making the code more expressive and easier to understand.
Thanks, and I’ll see you in the next tutorial.
Keep building!