In this tutorial, we will explore how to implement the main function in C++ within the context of robotics. The main function is the entry point for every C++ program, and understanding its structure and execution is important for any robotics engineer.
Prerequisites
- You have completed this tutorial: How to Organize Your C++ Code with std Namespace.
- I am assuming you are using Visual Studio Code, but you can use any code editor.
Directions
Open a terminal window, and type this:
cd ~/Documents/cpp_tutorial && code .
Create a new file, and save it as main_example.cpp. This will be our working file for this tutorial.
Type the following code into the editor:
#include <iostream>
int main(int argc, char *argv[]) {
std::cout << "Welcome to Robotics Bootcamp!" << std::endl;
// Print the command line arguments
std::cout << "Arguments passed to the program:" << std::endl;
for (int i = 0; i < argc; ++i) {
std::cout << i << ": " << argv[i] << std::endl;
}
return 0;
}
In this code, we start by including the <iostream> library to handle input and output operations.
Our main function here takes two parameters: argc and argv.
- argc is the count of command-line arguments passed to the program, including the program name
- argv is an array of character strings representing those arguments.
This setup allows your robot’s software to accept various startup parameters that could control different modes of operation or testing procedures.
When you run this program, you’ll see the welcome message followed by the list of command-line arguments.
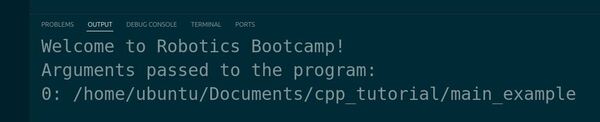
Try running your program from a terminal with additional arguments to see how it captures and displays them.
g++ main_example.cpp -o main_example
./main_example

Try running it with some command line arguments.
./main_example arg1 "argument 2" test123
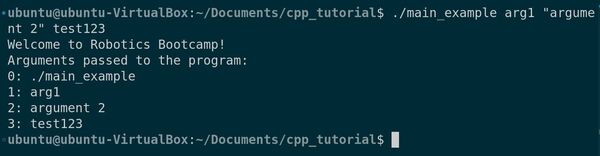
Thanks, and I’ll see you in the next tutorial.
Keep building!