In this tutorial, we will explore how to use cout and endl in C++ for outputting information in robotic projects. These elements are part of the iostream library and are essential for displaying messages and data in the console.
Prerequisites
- You have completed this tutorial: How to Organize Your C++ Code with std Namespace.
- I am assuming you are using Visual Studio Code, but you can use any code editor.
Directions
Open a terminal window, and type this:
cd ~/Documents/cpp_tutorial && code .
Let’s create a new C++ file and name it robot_output.cpp.
Type the following code into the editor:
#include <iostream>
using namespace std;
int main() {
string robot_status = "Active";
int x_position = 10;
int y_position = 20;
cout << "Robot Status: " << robot_status << endl;
cout << "Robot Position: (" << x_position << ", " << y_position << ")" << endl;
cout << "Initializing robot..." << endl;
// Simulating robot initialization
cout << "Robot initialized successfully!" << endl;
return 0;
}
In this code, we include the iostream header for input/output operations and use the using namespace std statement to bring the std namespace into the current scope.
Inside the main function, we declare variables for robot_status, x_position, and y_position, representing the status and position of the robot.
We use cout to output the robot’s status and position to the console.
The << operator is used to concatenate strings and variables.
The endl manipulator is used to insert a newline character and flush the output buffer.
Next, we simulate the robot initialization process by outputting messages using cout and endl.
Run the code.
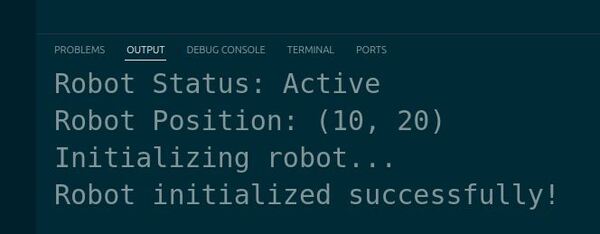
You should see the robot’s status, position, and initialization messages printed in the terminal.
That’s it. Thanks, and I’ll see you in the next tutorial.
Keep building!