In this tutorial, we are going to learn how to use threads in Python to perform multiple tasks simultaneously.
Prerequisites
- You have completed this tutorial: How to Use Advanced Object-Oriented Python.
Understanding Threads
Create a file called threading_example.py inside the following folder: ~/Documents/python_tutorial.
Now write the following code:
import threading
import time
def robot_task(name, duration):
print(f"{name} starting task.")
time.sleep(duration)
print(f"{name} has completed its task.")
# Creating threads
thread1 = threading.Thread(target=robot_task, args=("Robot 1", 2))
thread2 = threading.Thread(target=robot_task, args=("Robot 2", 3))
Here, we define a simple function robot_task that simulates a task by sleeping for a specified duration. Then, we create two threads, each assigned to execute this function.
Let’s complete the threading example by starting the threads and waiting for them to finish:
thread1.start()
thread2.start()
# Wait for both threads to complete
thread1.join()
thread2.join()
print("Both robots have completed their tasks.")
Now, run the script.
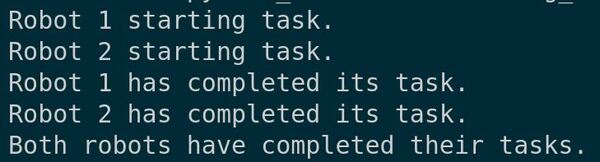
You should see output indicating that both robots are starting and completing their tasks, potentially overlapping in time, showing how threads allow simultaneous task execution.
Working with Regular Expressions
Let’s check out an indispensable tool for processing text data effectively—regular expressions, often abbreviated as regex.
Understanding how to use regular expressions will help you handle complex string manipulation tasks, which can be particularly useful in robotics for parsing logs, sensor data, or commands.
Create a new file named regex_examples.py inside the following folder: ~/Documents/python_tutorial.
First, we need to import the re module, which is Python’s library for handling regular expressions:
import re
Let’s define a function to demonstrate a basic regex operation—finding dates within a string. Imagine we’re processing a log file and we want to extract date entries formatted as dd-mm-yyyy.
def find_dates(text):
pattern = r'\b\d{2}-\d{2}-\d{4}\b'
dates = re.findall(pattern, text)
return dates
This function uses the re.findall() method, which searches through the text and returns all non-overlapping matches of the pattern described. Here, our pattern \b\d{2}-\d{2}-\d{4}\b looks for sequences that match the date format.
Let’s add a test case to use our function:
log_entry = "Error reported on 23-10-2023 at node 3. Previous error was on 01-10-2023."
found_dates = find_dates(log_entry)
print("Dates found:", found_dates)
Now, run the script.

You should see the output displaying the dates extracted from the string. This illustrates how regular expressions can simplify the task of searching and extracting data from text.
And that’s it for regular expressions.
Thanks, and I’ll see you in the next tutorial.
Keep building!