In this tutorial, we will explore how to use external libraries in C++ to enhance your robotics projects. External libraries provide pre-written code that can save you time and effort by offering ready-made solutions for common tasks.
Prerequisites
- You have completed this tutorial: Getting Started with C++ for Robotics Projects.
Directions
Open a terminal window, and type this:
cd ~/Documents/cpp_tutorial
code .
Now let’s install the Eigen library, a linear algebra library widely used in robotics for tasks such as matrix operations and transformations.
Open the integrated terminal in Visual Studio Code by going to ‘Terminal’ in the top menu and clicking on ‘New Terminal’.
In the terminal, type the following command to install Eigen:
sudo apt install libeigen3-dev
Enter your password if prompted and wait for the installation to complete.
Now, let’s create a new C++ file and name it simple_eigen_example.cpp.
Type the following code into the editor:
#include <iostream>
#include <eigen3/Eigen/Dense>
int main() {
Eigen::Vector3d v(1, 2, 3);
std::cout << "v = " << v.transpose() << std::endl;
return 0;
}
In this code, we include the necessary headers: iostream for input/output operations and Eigen/Dense for the Eigen library.
Then, in the main function, we create a 3D vector using Eigen’s Vector3d class and initialize it with values (1, 2, 3).
Finally, we print the transposed vector using std::cout.
Run the code.
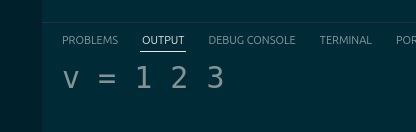
You should see the transposed vector printed in the terminal.
We could have also compiled the code this way:
g++ -I /usr/include/eigen3 simple_eigen_example.cpp -o simple_eigen_example
The “-I” flag in g++ tells the compiler where to look for additional header files/includes.
After successful compilation, run the executable with:
./simple_eigen_example
You should see the same transposed vector output as before.

That’s it! Keep building!