In this tutorial, we are going to explore how to accept user input in Python, an essential skill for programming interactive robots. Whether you’re controlling a robot remotely or setting up initial parameters, user input is key to dynamic interactions.
Prerequisites
- You have completed this tutorial: How to Use Python Range and Iterator Functions.
Accepting User Input
Let’s start by opening your code editor and creating a new file named robot_control_input.py inside the following folder: ~/Documents/python_tutorial.
First, we’ll create an interface for a user to input commands to control a robot.
# Command input for robot control
command = input("Enter command for the robot (move, stop, turn): ").lower()
if command == 'move':
print("Robot moving...")
elif command == 'stop':
print("Robot stopping...")
elif command == 'turn':
direction = input("Which direction to turn (left or right)? ").lower()
print(f"Robot turning {direction}")
else:
print("Unknown command.")
This script allows users to control basic robot movements via simple text commands.
Next, we might need to gather numerical data, such as setting a speed or defining a movement angle.
# Setting robot speed
speed = input("Enter robot speed (1-10): ")
speed = int(speed) # Convert the input from string to integer
print(f"Robot speed set to {speed}.")
Here, the user sets the robot’s speed, demonstrating how to handle numerical input.
Robots often work with sensors that require calibration or thresholds to be set by the user. Let’s simulate setting a sensor threshold.
# Setting sensor threshold
threshold = input("Set sensor threshold (e.g., temperature, distance): ")
threshold = float(threshold)
print(f"Sensor threshold set at {threshold} units.")
This example takes a threshold value for perhaps a temperature or distance sensor and applies it to the robot’s sensor settings.
We can also allow the user to input multiple configurations at once, useful for setting up initial parameters or during maintenance checks.
# Accepting multiple configuration values
configurations = input("Enter robot configurations separated by commas (arm length, camera resolution, wheel diameter, torso width): ")
config_list = configurations.split(',')
print("Robot configurations set:", config_list)
This allows the user to input a list of configuration values, which are then processed and applied to the robot.
Make sure to save your file. Now, let’s run the script to see how these input mechanisms work in action.
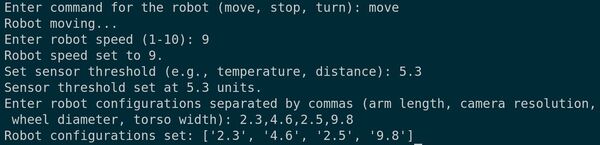
You’ll see how the program interacts with user commands and inputs, allowing for flexible and dynamic control of robot functions.
Using String Functions
Let’s explore string functions in Python and how they can be applied in robotic projects to manipulate and process textual data.
Strings in Python come with a variety of built-in functions that allow us to perform common operations.
Create a new file named robot_string_functions.py inside the following folder: ~/Documents/python_tutorial.
message = "Hello, World!"
print(len(message)) # Output: 13
print(message.upper()) # Output: HELLO, WORLD!
print(message.lower()) # Output: hello, world!
The len() function returns the length of the string, while upper() and lower() convert the string to uppercase and lowercase, respectively.
In robotic applications, string functions can be used to process and interpret textual commands or messages.
For example, let’s say we have a robot that receives commands in the format “COMMAND:PARAMETER”. We can use the split() function to extract the command and parameter.
command_str = "MOVE:FORWARD"
command, parameter = command_str.split(":")
print("Command:", command) # Output: MOVE
print("Parameter:", parameter) # Output: FORWARD
The split() function splits the string based on the specified delimiter (in this case, “:”) and returns a list of substrings.
String functions can also be used for data validation and cleaning. Let’s say we have a sensor that reads distance measurements as strings, but sometimes it includes unwanted characters.
distance_str = "12.5m"
distance_str = distance_str.strip("m")
distance = float(distance_str)
print("Distance:", distance) # Output: 12.5
The strip() function removes the specified characters from the beginning and end of the string, allowing us to extract the numeric value and convert it to a float.
Another useful string function is replace(), which replaces occurrences of a substring with another substring.
status_message = "Error: Sensor disconnected"
status_message = status_message.replace("Error", "Warning")
print(status_message) # Output: Warning: Sensor disconnected
This can be handy when processing log messages or status updates from robotic systems.
String functions offer a wide range of possibilities for manipulating and processing textual data in robotic applications. They can be used for parsing commands, extracting information from sensor readings, formatting messages, and much more.
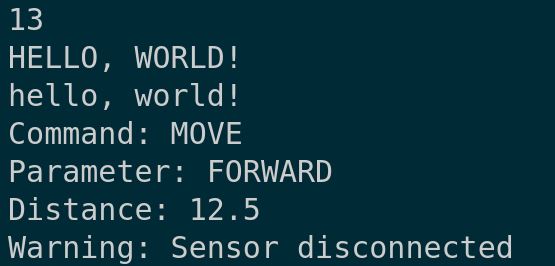
That’s it for this tutorial on using string functions in robotics. Thanks, and I’ll see you in the next tutorial.
Keep building!