In this tutorial, we are going to learn about how to handle exceptions in Python. Exception handling is important because it helps your programs to gracefully handle unexpected situations, like errors during execution, without crashing. This is particularly important in robotics, where an unhandled error can cause a robot to stop functioning or behave unpredictably.
Prerequisites
- You have completed this tutorial: How to Write Functions in Python.
When Things Go Wrong: Handling Exceptions
In Python, errors detected during execution are called exceptions. These might occur, for example, when trying to read a file that doesn’t exist or attempt to divide a value by zero. Without proper handling, these exceptions will cause your program to stop and raise an error message.
Let’s set up an example where errors might occur. We’ll attempt to divide two numbers, where the divisor is zero.
Open your code editor and create a new file named exception_handling.py inside the following folder: ~/Documents/python_tutorial.
def divide(x, y):
try:
result = x / y
print(f"The result is {result}")
except ZeroDivisionError:
print("You can't divide by zero!")
Here, the try block contains the code that might cause an exception. The except block tells Python what to do when a particular type of exception arises—in this case, a ZeroDivisionError.
You can catch different types of exceptions by specifying them explicitly, or catch all exceptions by just using except: without specifying an error type.
Now, let’s test our function with different inputs:
divide(10, 2) # This should work fine
divide(5, 0) # This should raise an exception
Run the script.
You should see the output showing the result of the first division and a message “You can’t divide by zero!” for the second. This demonstrates that our program can handle the ZeroDivisionError gracefully without crashing.

Now let’s try an advanced example. Create a file called advanced_exception_handling.py, and type the following code:
def divide_with_multiple_exceptions(x, y):
try:
# Convert inputs to float to handle string inputs
x = float(x)
y = float(y)
result = x / y
print(f"The result is {result}")
except ZeroDivisionError:
print("You can't divide by zero!")
except ValueError:
print("Please enter valid numbers!")
except Exception as e:
print(f"An unexpected error occurred: {e}")
# Test cases
print("Test 1: Normal division")
divide_with_multiple_exceptions(10, 2)
print("\nTest 2: Division by zero")
divide_with_multiple_exceptions(5, 0)
print("\nTest 3: Invalid input")
divide_with_multiple_exceptions("abc", 2)
print("\nTest 4: Float division")
divide_with_multiple_exceptions(10.5, 2.5)
This is an expanded version that shows how to handle multiple types of exceptions and includes more test cases. It:
- Handles ZeroDivisionError
- Handles ValueError for invalid inputs
- Includes a general Exception catch for unexpected errors
In robotics, exception handling is essential for maintaining robust and reliable control. For instance, if a sensor fails or provides invalid data, handling these exceptions can allow the robot to continue operating or switch to a safe state rather than failing outright.
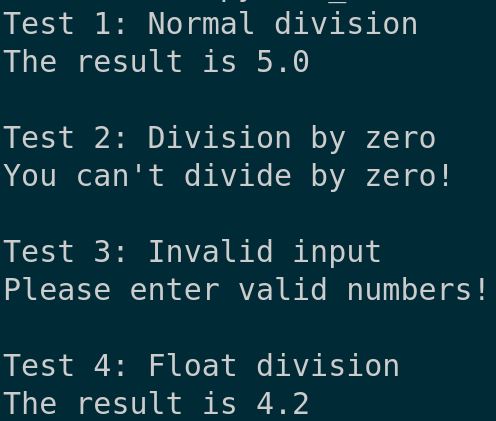
That’s it for this tutorial on handling exceptions. Thanks, and I’ll see you in the next tutorial.
Keep building!