In this tutorial, we are going to learn how to work with files in Python. Handling files is a fundamental skill in programming, essential for tasks like logging data, saving configurations, and processing input in robotics applications.
Prerequisites
- You have completed this tutorial: How to Create Classes and Objects in Python.
Working with Files: Create, Write, Close, Append, and Read
Let’s cover how to create, write, close, append, and read files using Python’s built-in functions.
Creating and Writing to a File
To create and write to a file, you can use Python’s open function with the ‘w’ mode. This mode creates the file if it does not exist and overwrites it if it does.
Create a file called file_handling.py inside the following folder: ~/Documents/python_tutorial.
Write the following code:
file = open('example.txt', 'w')
file.write('Hello, world!')
file.close()
It’s important to close the file when you’re done with it. Closing the file ensures that all changes are saved and frees up system resources.
Appending to a File
If you want to add content to a file without overwriting the existing content, you use the ‘a’ mode with the open function.
file = open('example.txt', 'a')
file.write('\nAdding a second line.')
file.close()
Reading from a File
To read the contents of a file, you use the ‘r’ mode with open. There are several methods to read, such as read(), readline(), which reads one line at a time, and readlines(), which returns a list of lines.
file = open('example.txt', 'r')
content = file.read()
print(content)
file.close()
Using the with Statement
While using open() and close(), it’s safer to handle files with a context manager using the with statement. This ensures that the file is properly closed after its suite finishes, even if an exception occurs.
with open('example.txt', 'r') as file:
content = file.read()
print(content)
You should see the content of example.txt printed, which now includes both the original line and the appended line.
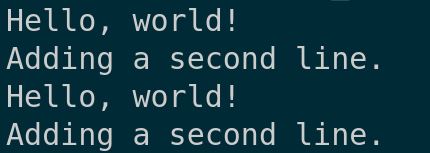
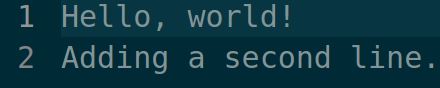
In robotics, file handling is important for tasks such as storing sensor readings, saving configuration settings, or logging events for diagnostics. Efficient file management can help maintain the integrity and performance of robotic systems.
That’s it for this tutorial on working with files in Python. Thanks, and I’ll see you in the next tutorial.
Keep building!