In this tutorial, we are going to learn how to use iterators in Python, which allow us to traverse through sequences of elements efficiently.
Prerequisites
- You have completed this tutorial: How to Write Loops in Python.
Working with Iterators
Create a program called working_with_iterators.py inside the following folder: ~/Documents/python_tutorial.
Let’s create a list and an iterator from it using the iter() function.
my_list = [1, 2, 3, 4, 5]
my_iterator = iter(my_list)
We can traverse the iterator using the next() function.
print(next(my_iterator)) # Output: 1
print(next(my_iterator)) # Output: 2
print(next(my_iterator)) # Output: 3
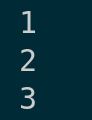
Alternatively, we can use a for loop to iterate through the elements automatically.
my_iterator = iter(my_list) # Reset the iterator
for item in my_iterator:
print(item)
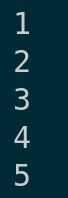
It’s important to note that when there are no more elements in the iterator, a StopIteration exception is raised, signaling the end of the iteration.
Iterators are fundamental for efficient data processing in Python and are particularly useful in robotic projects when working with large datasets or sequences of data.
Working with Ranges
Let’s dive into the concept of ranges in Python, which provide a convenient way to generate sequences of numbers.
Create a program called working_with_ranges.py inside the following folder: ~/Documents/python_tutorial.
Let’s start by creating a simple range using the range() function.
my_range = range(5)
print(my_range) # Output: range(0, 5)
The range() function generates a sequence of numbers starting from 0 (by default) and ending at the specified number (exclusive).
We can convert the range to a list to see its elements.
my_list = list(my_range)
print(my_list) # Output: [0, 1, 2, 3, 4]

The range() function can also take additional arguments to specify the start value and the step size.
my_range = range(2, 10, 2)
my_list = list(my_range)
print(my_list) # Output: [2, 4, 6, 8]

Here, the range starts from 2, ends at 10 (exclusive), and increments by 2 in each step.
Ranges are commonly used in for loops to iterate a specific number of times.
for i in range(5):
print(i)
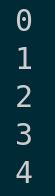
This loop iterates 5 times, with the variable i taking values from 0 to 4.
Ranges are memory-efficient because they generate numbers on-the-fly rather than storing them in memory. This makes them useful when working with large sequences of numbers.
In robotic projects, ranges can be used for tasks like iterating over a sequence of angles for a robotic arm or generating a series of time steps for a simulation.
That’s it for this tutorial on ranges. Thanks, and I’ll see you in the next tutorial.
Keep building!